辞書 dict から JSON に変換して JSON から dict に戻す
下記のコードは、辞書 dict から JSON に変換して、その JSON を辞書に戻すサンプルです。
dict のメンバには、ndarray のデータを仕込むことはできないので、いったん tolist() メソッドを使って list に変換してから仕込みます。
dict のメンバからデータを取り出すときは、当然ながら list ですので、それを ndarray に変換する場合は np.array() メソッドを用います。
大まかには dict0 → the_json → dict1 というコードの流れです。
import json
import numpy as np
from PIL import Image
import matplotlib.pyplot as plt
KEY_00 = "ImageWidth"
KEY_01 = "ImageHeight"
KEY_02 = "Image"
# ndarray を確保する.
w0 = 512
h0 = 256
num0 = w0 * h0
data0 = np.zeros( num0, dtype = np.uint8 )
# ndarray にデータを仕込む.
for j in range( h0 ):
adrs = w0 * j
for i in range( w0 ):
data0[adrs] = i + j
adrs += 1
# 辞書型に ndarray は仕込めないのでリストに変換する.
list0 = data0.tolist()
# 辞書型にデータを登録する.
the_dict0 = dict()
the_dict0[KEY_00] = w0
the_dict0[KEY_01] = h0
the_dict0[KEY_02] = list0
# 辞書型からJSON文字列へ.
the_json = json.dumps( the_dict0, indent = 4, ensure_ascii = True )
# JSON文字列から辞書型へ.
the_dict1 = json.loads( the_json )
# 辞書からデータを取り出す.
w1 = the_dict1[KEY_00]
h1 = the_dict1[KEY_01]
list1 = the_dict1[KEY_02]
# リストから ndarray へ.
data1 = np.array( list1 )
# ndarrayを2次元化する.
data1 = data1.reshape( h1, w1 )
# 念のため ndarray を 0 ~ 255 にする.
data1 = data1.astype( np.uint8 )
# ndarray から Pillow イメージに変換する.
im = Image.fromarray( data1 )
# Matplotlib で画像を表示する.
plt.imshow( im, cmap='gray')
plt.show()
print( "finish." )
実行すると、下記のような matplotlib のグラフを表示します。
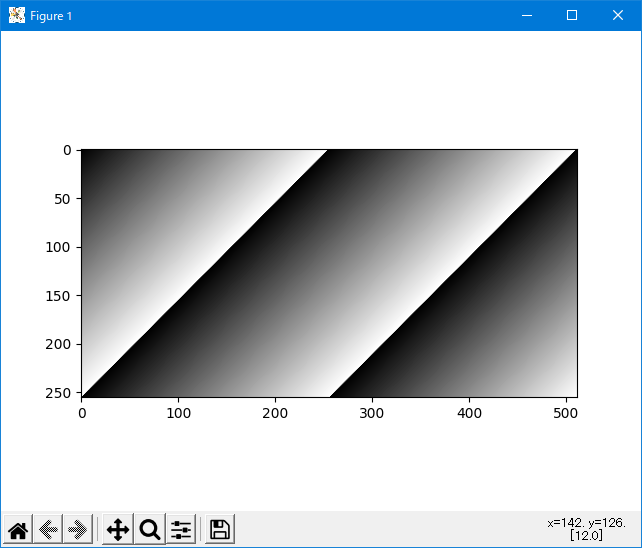