特定のビットを反転する
特定のビットを反転するには ^= を使います。
// ビット0 (LSB) だけを反転する.
Flags ^= 0x00000001;
// ビット1だけを反転する.
Flags ^= 0x00000002;
// ビット2だけを反転する.
Flags ^= 0x00000004;
こんな感じのアプリケーションを作る場合は
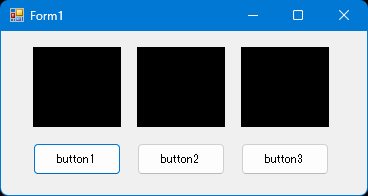
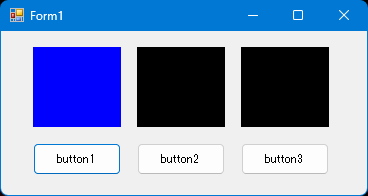
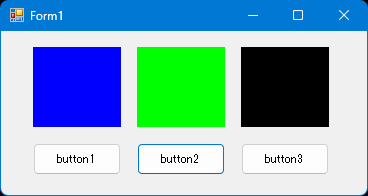
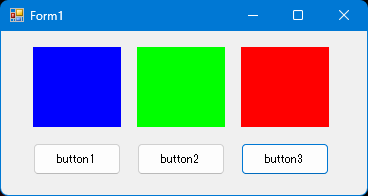
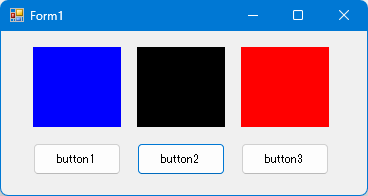
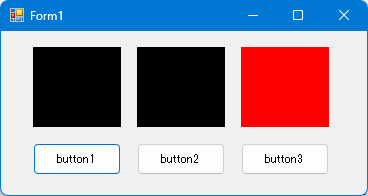
下記のコードになります。
using System;
using System.Drawing;
using System.Windows.Forms;
namespace aaa
{
public partial class Form1 : Form
{
const uint BIT_R = 0x00000001;
const uint BIT_G = 0x00000002;
const uint BIT_B = 0x00000004;
uint Flags;
public Form1()
{
InitializeComponent();
}
private void Form1_Load( object sender, EventArgs e )
{
Flags = 0x00000000;
}
private void button1_Click( object sender, EventArgs e )
{
Flags ^= BIT_B;
this.Invalidate( true );
}
private void button2_Click( object sender, EventArgs e )
{
Flags ^= BIT_G;
this.Invalidate( true );
}
private void button3_Click( object sender, EventArgs e )
{
Flags ^= BIT_R;
this.Invalidate( true );
}
private void pictureBox1_Paint( object sender, PaintEventArgs e )
{
Color c;
if (( Flags & BIT_B ) == BIT_B )
{
c = Color.Blue;
}
else
{
c = Color.Black;
}
pictureBox1.BackColor = c;
}
private void pictureBox2_Paint( object sender, PaintEventArgs e )
{
Color c;
if (( Flags & BIT_G ) == BIT_G )
{
c = Color.Lime;
}
else
{
c = Color.Black;
}
pictureBox2.BackColor = c;
}
private void pictureBox3_Paint( object sender, PaintEventArgs e )
{
Color c;
if (( Flags & BIT_R ) == BIT_R )
{
c = Color.Red;
}
else
{
c = Color.Black;
}
pictureBox3.BackColor = c;
}
}
}