定義済み色をリストアップする
あらかじめ名前で定義されている色、たとえば Color.Aqua や Color.Orange など、そういった色を列挙する方法を紹介します。また、その色の RGB 成分を参照する方法も同時に紹介します。
Fig. 1 に示すアプリケーションを作って確認します。
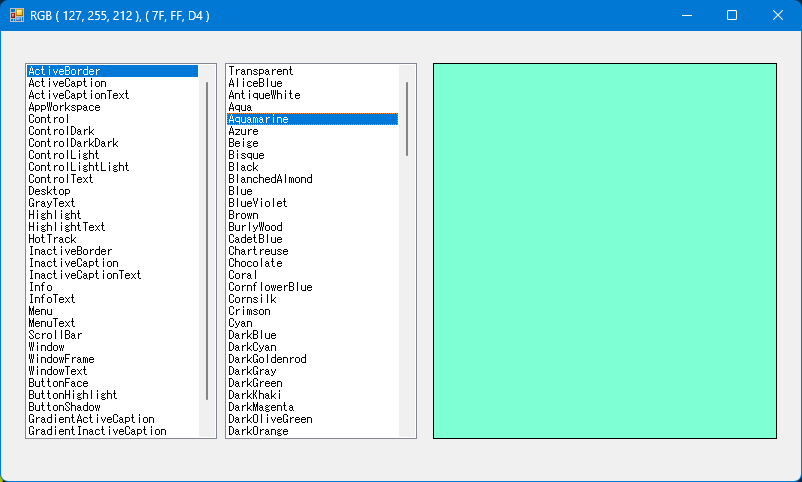
Form1 に下記のコントロールを配置してください。
listBox1 という名前の ListBox
listBox2 という名前の ListBox
pictureBox1 という名前の PictureBox
Form には Load イベントを定義してください。
ListBox には SelectedIndexChanged イベントを定義してください。
PictureBox には Paint イベントを定義してください。
具体的には、下記のコードです。22行目で定義済み色の配列を取得しています。
その色がシステムカラーかどうかは IsSystemColor というプロパティの true/false で知ることができます。色の名前から Color を取得するには Color.FromName() というメソッドを利用します。
using System;
using System.Drawing;
using System.Windows.Forms;
namespace aaa
{
public partial class Form1 : Form
{
// ピクチャボックスを塗りつぶす色.
Color TheColor;
public Form1()
{
InitializeComponent();
}
private void Form1_Load( object sender, EventArgs e )
{
// すべての定義済み色を列挙した配列を取得する.
Array arr = Enum.GetValues( typeof( KnownColor ));
// いったんリストボックスのアイテムをクリアする.
listBox1.Items.Clear();
listBox2.Items.Clear();
// リストボックス項目に仕込む.
for ( int k = 0; k < arr.Length; k++ )
{
KnownColor kc = (KnownColor)( arr.GetValue(k));
Color c = Color.FromKnownColor( kc );
if ( c.IsSystemColor )
{
// システムカラーの場合.
listBox1.Items.Add( c.Name );
}
else
{
// そうでないカラーの場合.
listBox2.Items.Add( c.Name );
}
}
// それぞれ何色あるか起動時に表示する.
// StringBuilder sb = new StringBuilder();
// sb.AppendLine( String.Format( "システムカラー {0} 色.", listBox1.Items.Count ));
// sb.AppendLine( String.Format( "そうでないカラー {0} 色.", listBox2.Items.Count ));
// MessageBox.Show( sb.ToString().Trim());
}
private void listBox1_SelectedIndexChanged( object sender, EventArgs e )
{
int k = listBox1.SelectedIndex;
String color_name = listBox1.Items[k].ToString();
// 色の名前から Color を取得する.
TheColor = Color.FromName( color_name );
// ピクチャボックスの再描画を要請する.
pictureBox1.Invalidate( true );
}
private void listBox2_SelectedIndexChanged( object sender, EventArgs e )
{
int k = listBox2.SelectedIndex;
String color_name = listBox2.Items[k].ToString();
// 色の名前から Color を取得する.
TheColor = Color.FromName( color_name );
// ピクチャボックスの再描画を要請する.
pictureBox1.Invalidate( true );
}
private void pictureBox1_Paint( object sender, PaintEventArgs e )
{
int pw = pictureBox1.Width;
int ph = pictureBox1.Height;
// ピクチャボックス全体を指定の色で塗りつぶす.
using ( SolidBrush sb = new SolidBrush( TheColor ))
{
e.Graphics.FillRectangle( sb, 0, 0, pw, ph );
}
// ピクチャボックスの枠線は常に黒とする.
using ( Pen pen = new Pen( Color.Black ))
{
int xs = 0;
int ys = 0;
int xe = pw - 1;
int ye = ph - 1;
e.Graphics.DrawLine( pen, xs, ys, xe, ys );
e.Graphics.DrawLine( pen, xe, ys, xe, ye );
e.Graphics.DrawLine( pen, xe, ye, xs, ye );
e.Graphics.DrawLine( pen, xs, ye, xs, ys );
}
// 3原色に分解する.
byte r = TheColor.R;
byte g = TheColor.G;
byte b = TheColor.B;
// 10進と16進で文字列を生成する.
String str0 = String.Format( "( {0}, {1}, {2} )", r, g, b );
String str1 = String.Format( "( {0:x}, {1:x}, {2:x} )", r, g, b );
// タイトルバーに表示する.
str1 = str1.ToUpper();
this.Text = String.Format( "RGB {0}, {1}", str0, str1 );
}
}
}