ファイルを開く保存ダイアログ(Windows Forms)
ユーザが選択したファイルのパスを取得するには OpenFileDialog クラスを使います。保存するファイルのパスをユーザに入力させるには SaveFileDialog クラスを使います。 みなさんご存知の下記のダイアログです。
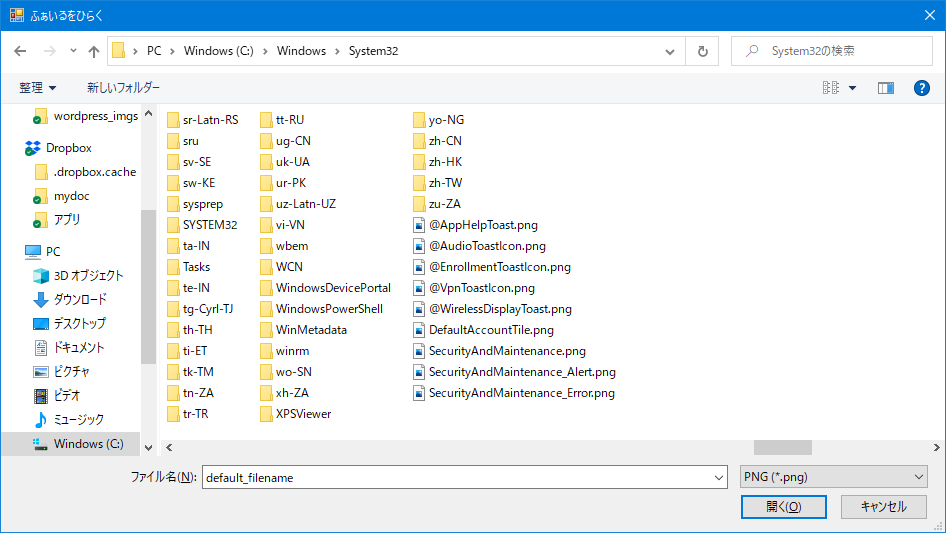
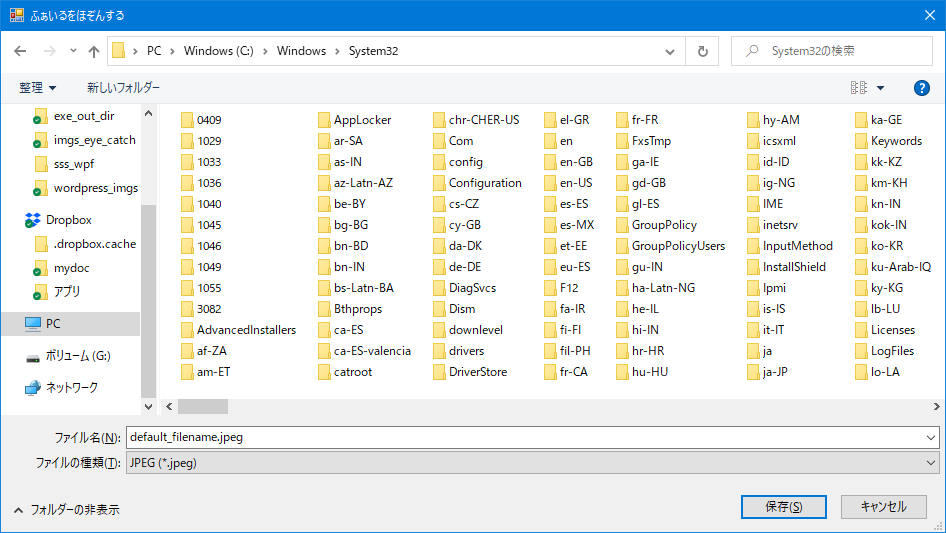
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace aaa
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void menuFileOpen_Click( object sender, EventArgs e )
{
OpenFileDialog ofd = new OpenFileDialog();
// ダイアログのタイトル.
ofd.Title = "ふぁいるをひらく";
// 拡張子フィルタ.
ofd.Filter = "Bitmap|*.bmp|JPEG|*.jpeg|PNG|*.png|ALL|*.*";
// 基数は1.
ofd.FilterIndex = 3;
// デフォルト表示ファイル名.
ofd.FileName = "default_filename";
// ダイアログを表示する.
DialogResult dr = ofd.ShowDialog();
// 戻り値を評価する.
if ( dr == DialogResult.Cancel )
{
MessageBox.Show( "Canceled." );
}
else
{
String filepath = ofd.FileName;
MessageBox.Show( filepath );
}
}
private void menuFileSave_Click( object sender, EventArgs e )
{
SaveFileDialog sfd = new SaveFileDialog();
// ダイアログのタイトル.
sfd.Title = "ふぁいるをほぞんする";
// 拡張子フィルタ.
sfd.Filter = "Bitmap|*.bmp|JPEG|*.jpeg|PNG|*.png|ALL|*.*";
// 基数は1.
sfd.FilterIndex = 2;
// デフォルト表示ファイル名.
sfd.FileName = "default_filename";
// ダイアログを表示する.
DialogResult dr = sfd.ShowDialog();
// 戻り値を評価する.
if ( dr == DialogResult.Cancel )
{
MessageBox.Show( "Canceled." );
}
else
{
String filepath = sfd.FileName;
MessageBox.Show( filepath );
}
}
}
}
このページは Windows Forms アプリケーションで OpenFileDialog, SaveFileDialog を使う紹介でした。WPF アプリケーションで同様のアイテムを使う方法は下記の記事で紹介しています。
ファイルを開く保存ダイアログ(WPF)
ファイルを開くダイアログは OpenFileDialog、保存は SaveFileDialog を使います。WindowsForms のときと微妙に違う点を含め解説します。