ファイルを開く保存ダイアログ(WPF)
ユーザが選択したファイルのパスを取得するには OpenFileDialog クラスを使います。保存するファイルのパスをユーザに入力させるには SaveFileDialog クラスを使います。みなさんご存知の下記のダイアログです。
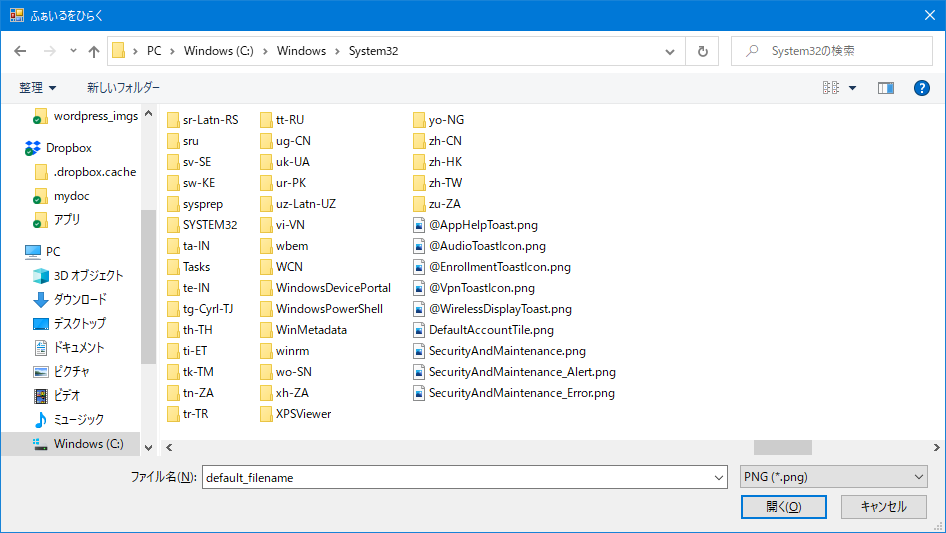
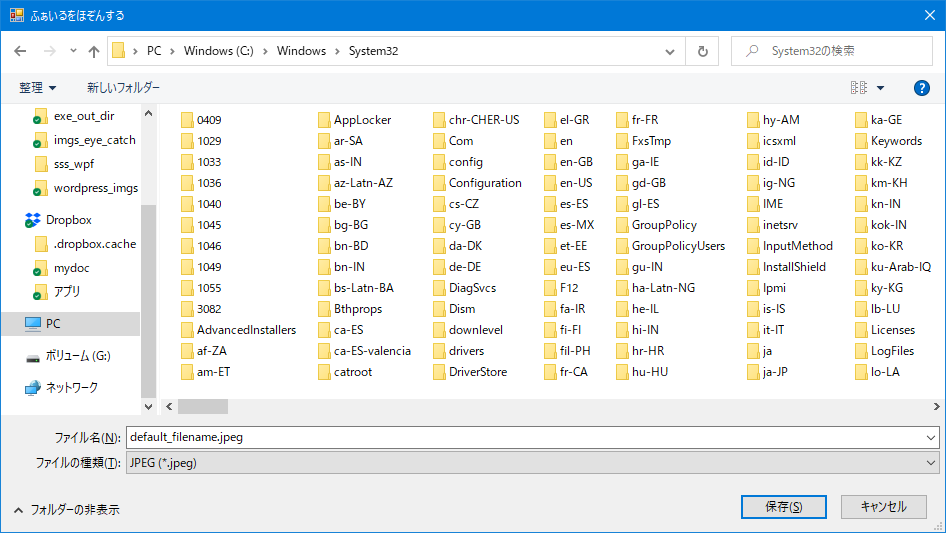
Windows Formsのアプリケーションでは
- System.Windows.Forms.OpenFileDialog
- System.Windows.Forms.SaveFileDialog
でしたが、
WPFのアプリケーションでは
- Microsoft.Win32.OpenFileDialog
- Microsoft.Win32.SaveFileDialog
を使います。
どちらもダイアログ表示には同名のメソッド ShowDialog を使いますが、WPF では ShowDialog の戻り値が bool? 型であることに注意してください。( bool? 型は bool 型とは微妙に違う型です )
下記の名前空間の追加をお忘れなく.
using Microsoft.Win32;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Navigation;
using System.Windows.Shapes;
using System.Windows.Media;
// この using 追加を忘れずに. Don't forget to add this sentence.
using Microsoft.Win32;
namespace aaa
{
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
private void menuFileOpen_Click( object sender, RoutedEventArgs e )
{
OpenFileDialog ofd = new OpenFileDialog();
// ダイアログのタイトル.
ofd.Title = "ふぁいるをひらく";
// 拡張子フィルタ.
ofd.Filter = "Bitmap|*.bmp|JPEG|*.jpeg|PNG|*.png|ALL|*.*";
// 基数は1.
ofd.FilterIndex = 3;
// デフォルト表示ファイル名.
ofd.FileName = "default_filename";
// 戻り値は bool ではなくて bool? であることに注目.
bool? ret = ofd.ShowDialog();
// bool ではないので if ( ret ) にはできない.
if ( ret == true )
{
String filepath = ofd.FileName;
MessageBox.Show( filepath );
}
else
{
MessageBox.Show( "Canceled." );
}
}
private void menuFileSave_Click( object sender, RoutedEventArgs e )
{
SaveFileDialog sfd = new SaveFileDialog();
// ダイアログのタイトル.
sfd.Title = "ふぁいるをほぞんする";
// 拡張子フィルタ.
sfd.Filter = "Bitmap|*.bmp|JPEG|*.jpeg|PNG|*.png|ALL|*.*";
// 基数は1.
sfd.FilterIndex = 2;
// デフォルト表示ファイル名.
sfd.FileName = "default_filename";
// 戻り値は bool ではなくて bool? であることに注目.
bool? ret = sfd.ShowDialog();
// bool ではないので if ( ret ) にはできない.
if ( ret == true )
{
String filepath = sfd.FileName;
MessageBox.Show( filepath );
}
else
{
MessageBox.Show( "Canceled." );
}
}
}
}
このページは WPF アプリケーションで OpenFileDialog, SaveFileDialog を使う紹介でした。Windows Forms アプリケーションで同様のアイテムを使う方法は下記の記事で紹介しています。